MVC Pattern
Scaffolding your project
If we wanted, we could build our entire application inside of that single barebones.py file. But that would become a massive file and very hard to maintain. So instead, we are going to break out all of the different parts. But first, lets talk about the Model View Controller, or MVC, Pattern.
The MVC Pattern is very commonly used in web development. It is used to keep the different components separate and modularized. Let's go through the three parts
Goal
By the end of this section, you should be able to understand the changes made in Checkpoint 2 - Scaffolded.
Model
The model is your blueprint for how to structure all of your variables. If you're just building a static website or some kind of portfolio, you won't need a model. But if you want to build a blog or some application where you can upload photos or add new content, you will need to construct a model. The model is essentially just the Classes or objects that your app will use. If you are familiar with databases, the model is used to lay out the database and how values will be organized in the database tables. We will go into more detail later because we won't actually be using one just yet.
View
The Views are the HTML pages that the user will see and interact with. Generally, these HTML pages will show the data that is being stored in the Model and provide some way for the user to create, read, update, or delete the data. However, some of the pages can be static and don't need to display any data from the Model.
Controller
The Controllers are where most of the logic happens. They directly of indirectly manage the data that each view displays and then how any new or updated data translates to the Model.
Interaction Pipeline
Let's go through an example to illustrate how these three separate parts fit together. Let's assume we have a blogging app and a user wants to add a comment to a blog post. Here's how I like to think about the interaction pipeline:
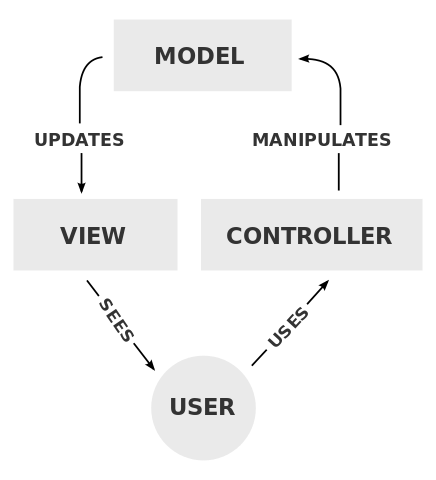
- Using a routing process, a specific URL is tied to a specific Controller function
- The correct Controller function performs the necessary logic, initializes the correct variables, and loads required values from the Model
- This data is passed to the View by the Controller in order to render a page for the user to interact with
- The user will submit a comment which is passed back to the Controller for that View
- The Controller will then save the user's comment back to the Model to be loaded again the next time
Let the Games Begin
So we want to to break out our current codebase into something that resembles and MVC Pattern. Right now, we have all of our necessary code in one file: barebone.py. Let's start restructuring our directory to easily add in MVC components.
Open up Checkpoint 2 - Scaffolded so that you can examine the new project structure and follow along while I outline what each file does and why the changes were made.
You'll notice there are a handful more files and folders, but about the same amount of code. All of the code has been broken out of barebones.py into separate files or even separate directories. Lets go through each item.
controllers/__init__.py
This file is empty. The purpose that this file serves is to let the Python language know that the 'controllers' directory contains more Python files.
controllers/base.py
This file contains the "master controller" class. All of the other controllers will inherit this controller so that code doesn't have to be duplicated. It sets up the Jinja2 environment, which is what helps generate the Views, as well as passing any variables you want to the View to be displayed.
controllers/home.py
This is the controller for the home.html view. As you can see, it's much more simple than the base.py
controller, but it inherits all of the functionality with (BaseRequestHandler)
. And
using the generate()
function, it will render the home.html
view and
pass along a variable called "test".
controllers/second.html
This is the controller for the second.html view. It's just like the home controller, only it renders a different view with different variables.
static/
This directory will contain all of the CSS, JavaScript, and images that your application might use. But we are not going to cover this in too much detail right now. It's mostly just a placeholder.
views/base.html
Just like controller/base.py, views/base.html takes care of a lot of the boiler plate code that goes into HTML pages. You can just extend this file with all of your other Views and not have to worry about copying all of the code already in this file.
views/home.html
This is a much smaller HTML file because it's extending the base.html file. If you look at the base.html
file and the home.html file side-by-side, you can image just copying and pasting everything inside of the
corresponding {% block %}
statements back into the base.html file. Not all of the
block statements need to be filled. Notice how home.html uses the 'title' and 'body' blocks but not the
'head' block.
views/second.html
This HTML file looks almost identical to the home.html file, except it has a different title.
app.yaml
This is the configuration file for the app. Notice how is now using the Jinja library to generate the HTML pages.
models.py
This is a placeholder for where we will put our model, which we'll cover later.
setting.py
This is for general settings or variables that you want to be globally available in all Views. Make
sure to update the values
dict in controllers/base.py with anything extra you add here.
urls.py
This is the routing mechanism and is very important. It tells your application which Controller to use depending on the URL. Right now, we have the root address '/' going to the Home controller, and '/two' pointing to the Second controller.