Design
Bootstrappin' teh stylez
Now it's time to talk more about how your site looks. We've set up a very simple back
end, now it's time to work more on the front end. Remember that
static/
directory that we setup as a placeholder? Lets start loading it up with some useful
CSS and JavaScript.
Remember, HTML is the code that's necessary for putting text and content on your page, but CSS is necessary for making it look goood. It's used to change things from the margins of your document to the color and font of your text. Once we're happy with our HTML and CSS, we'll add in some JavaScript. JavaScript is used to make things happen on the webpage. If you click a dropdown button, a menu should appear - this is done using JavaScript.
Goal
By the end of this section, you should be able to understand the changes made in Checkpoint 3 - Bootstrapped.
HTML with Jinja
The most fundamental aspect of any website is the HTML. But HTML is static, meaning that it doesn't ever
change. You can't have logic in your HTML to make things dynamic (like if
statements in
Python). You can't use variables in HTML to keep track of the active user's username, for example. But
there is a nifty templating language called Jinja that allows you to embed some Python into your HTML
pages (this is why there was some Jinja code in controllers/base.py). I highly recommend looking through
the Jinja Documentation while you're figuring out how to
build your webpages.
If you want access to the variables in your Python Controller from your Jinja View, make sure to pass the
variables into the self.generate()
call at the end of each Controller function.
Let's go though an example of how to pass variables from a Controller to a View and how to display them with HTML and Jinja.
controllers/about.py
from controllers.base import BaseRequestHandler
import random
class About(BaseRequestHandler):
def get(self):
name = "Teddy Roosevelt"
random_int = random.randint(0, 100) # generate random number between 0 and 100
if random_int % 2 == 0: # if random number is even
address = "1600 Pennsylvania Ave.\nNorthwest, Washington, DC 20500"
age = 43
else: # if random number is odd
address = "The Moon"
age = 51
self.generate("about.html", {
"name": name,
"address": address,
"age": age
})
views/about.html
{% extends "base.html" %}
{% block title %}About{% endblock %}
{% block body %}
<h2>About</h2>
<p>
Welcome to the about page. My name is {{ name }}. I am {{ age }}
years old and I live at this address:
{{ address }}
</p>
{% endblock %}
And through the magic of Jinja, a full HTML page will be created that contains the variables you passed to it from the controller.
Bootstrap
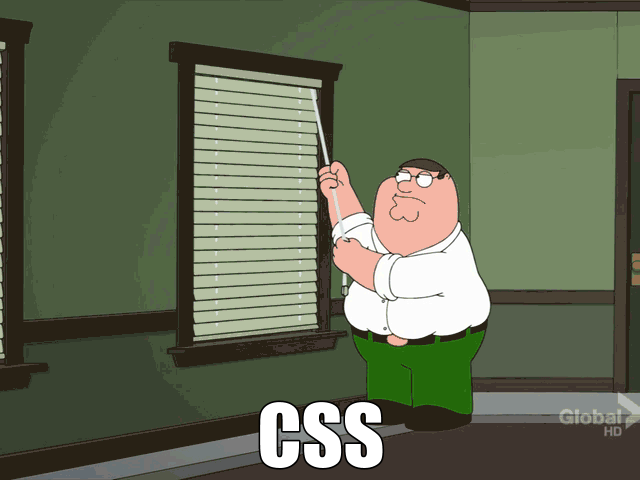
CSS can become an unweildy beast. I feel that this GIF perfectly reflects my experience with it. Thankfully, the good folks at Twitter created a library of sorts for including CSS in your project. You don't have to worry about writing any of your own CSS, you just have to make sure you give certain elements the correct class names. However, if you want to customize it and write some of your own CSS, you can tweak the styles however you want. The Bootstrap Documentation is very helpful.
JavaScript
Alright, so you've got the tools to put dynamic content on your page before it loads, the tools to design everything to look awesome, but now you want to make the pages dynamic after it loads. JavaScript is powerful and can be used to build entire web apps, but we will only be using it at a very basic level.
So what might we want to do with JavaScript? Well, lets do one of the most basic things that can be done, making something appear when something else is clicked (just like the GIF I related to CSS earlier (ahh, full circle)).
Let's create the file static/js/show.js
and write a small amount of JavaScript. This function
will take an element's ID, and then unhide it by removing the 'hide' class.
function show(elementId) {
var ele = document.getElementById(elementId);
ele.className = ele.className.replace("hide", "");
}
Now, let's tweak views/about.html
to take advantage of this JavaScript function we just wrote.
Let's also add a few CSS classes from Bootstrap to our page.
{% extends "base.html" %}
{% block head %}
<script src="/static/js/show.js"></script>
{% endblock %}
{% block title %}About{% endblock %}
{% block body %}
<h2>About</h2>
<p class="col-md-3">
Welcome to the about page. My name is {{ name }}. I am {{ age }}
years old. Click <a href="#" onClick="show('address')">here to see my address</a>
</p>
<p class="hide" id="address">
{{ address }}
</p>
{% endblock %}